Learn marketable open source skills
76% of organizations are unable to find enough individuals with open source skills. You can take advantage by learning these high-demand skills and build your successful career.
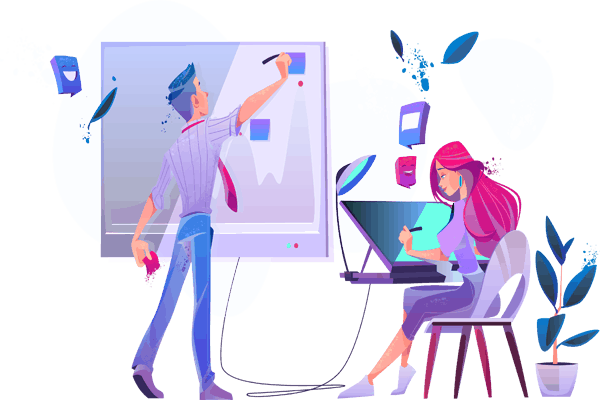
100’s of Tutorials
Build your modern Linux skills with our comprehensive tutorial library
Expand your Linux System Administration and Engineering skills with our constantly updated tutorial library. Whether you’re just finding your topics, subjects, or any open-source tools, prepping for expertise in those skills, or looking to go deep into specialty topics like serverless and machine learning, we’ve got you covered.
Ubuntu
Learn Linux administration with Ubuntu Operating System.
Bash Script
Learn admin task automation using bash scripts.
Apache HTTP
Learn Apache webserver to host your website or web apps.
KVM
Learn server virtualization technology with KVM.
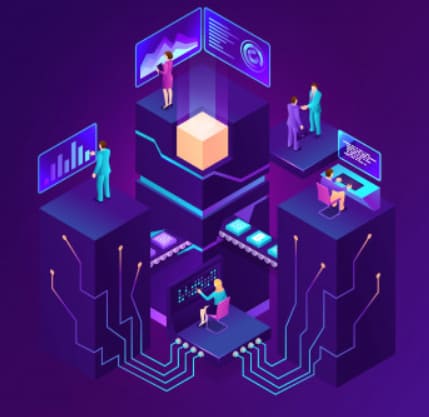
Become an expert
Be an expert, master in modern Linux and opensource technologies, and level up your career – whether you’re starting or a seasoned pro. Learn by doing with LinuxConcept.
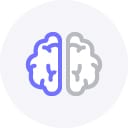
Learn by Doing
Learn fast, even faster.
Linux Concept provides multiple resources for study and learns Linux on a fast track. We have numerous study sections, including articles, tutorials, guides, documents, ppt, video, etc.
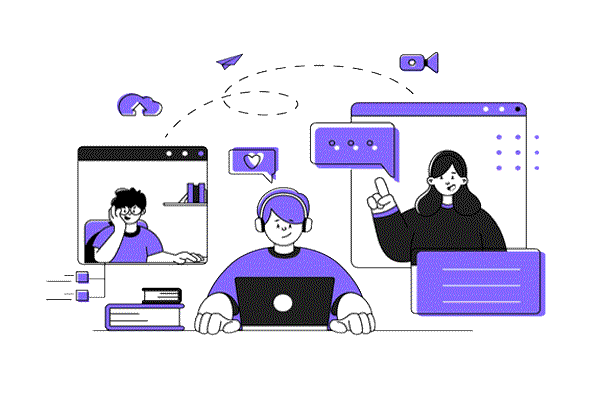
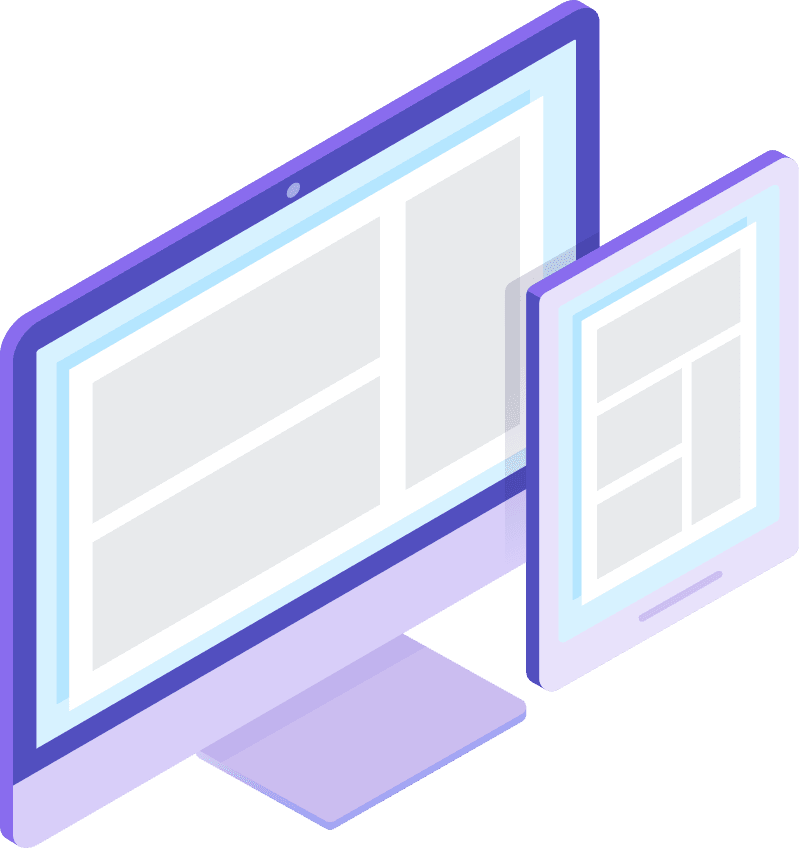

Build your portfolio
Expert the skills to drive your career smartly and successful
With the number of tutorials, guides, docs, ppts, audio, video, we give you plenty of room to build your portfolio whether you’re accelerating your career and skilling up knowledge.
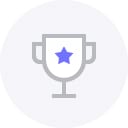
Achieve your goals
Set the Goal, Crack it.
Using our tutorial, guide, docs, audio, video, you can make your own goal to learn the skills and achieve them to build your successful career.
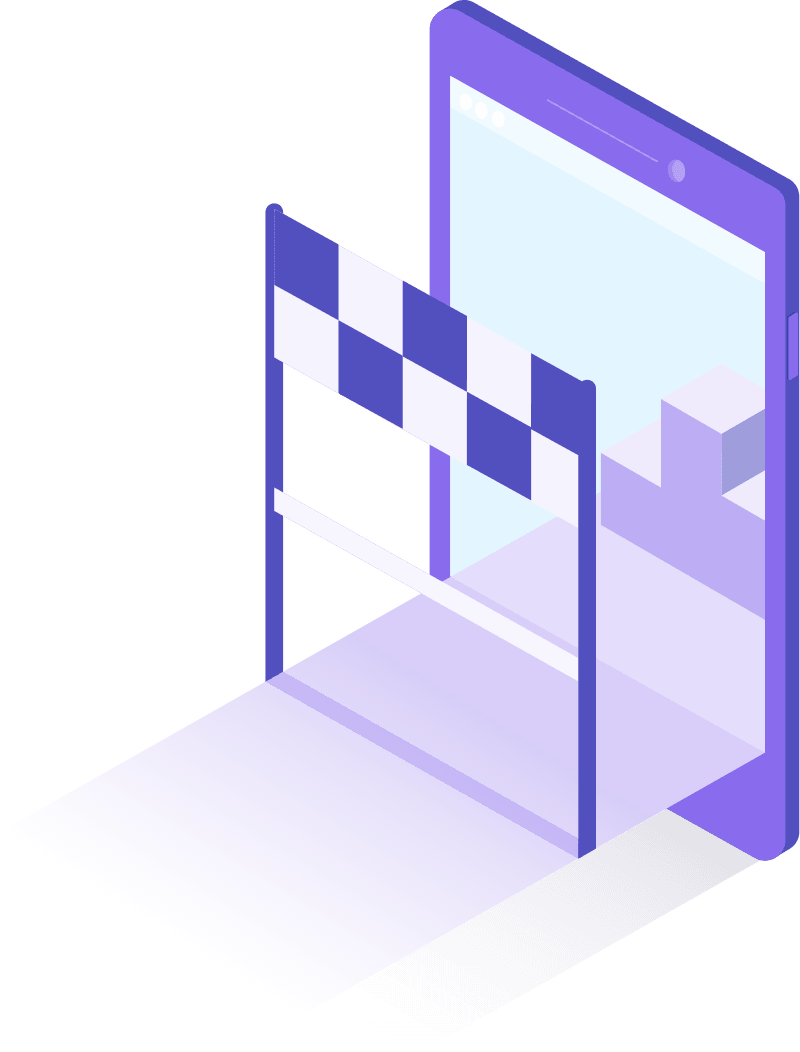